Bluetooth機器の検出
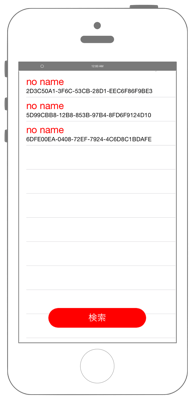
Swift 4.0
import UIKit
import CoreBluetooth
class ViewController: UIViewController {
var tableView: UITableView!
var uuids = Array<UUID>()
var names = [UUID : String]()
var peripherals = [UUID : CBPeripheral]()
var centralManager: CBCentralManager!
let button = UIButton()
override func viewDidLoad() {
super.viewDidLoad()
let barHeight = UIApplication.shared.statusBarFrame.size.height
let displayWidth = self.view.frame.width
let displayHeight = self.view.frame.height
tableView = UITableView(frame: CGRect(x: 0, y: barHeight, width: displayWidth, height: displayHeight - barHeight))
tableView.register(UITableViewCell.self, forCellReuseIdentifier: "MyCell")
tableView.dataSource = self
tableView.delegate = self
self.view.addSubview(tableView)
button.frame = CGRect(x: 0, y: 0, width: 200, height: 40)
button.backgroundColor = UIColor.red
button.layer.masksToBounds = true
button.setTitle("検索", for: UIControlState.normal)
button.setTitleColor(UIColor.white, for: UIControlState.normal)
button.layer.cornerRadius = 20.0
button.layer.position = CGPoint(x: self.view.frame.width/2, y:self.view.frame.height-50)
button.tag = 1
button.addTarget(self, action: #selector(onClickMyButton(sender:)), for: .touchUpInside)
self.view.addSubview(button);
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
@objc func onClickMyButton(sender: UIButton){
self.uuids = []
self.names = [:]
self.peripherals = [:]
centralManager = CBCentralManager(delegate: self, queue: nil, options: nil)
}
}
extension ViewController: UITableViewDataSource{
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.names.count
}
}
extension ViewController: UITableViewDelegate{
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let uuid = self.uuids[indexPath.row]
print("Num: \(indexPath.row)")
print("uuid: \(uuid.description)")
print("Name: \(String(describing: self.names[uuid]?.description))")
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = UITableViewCell(style: UITableViewCellStyle.subtitle, reuseIdentifier:"MyCell" )
let uuid = self.uuids[indexPath.row]
cell.textLabel!.sizeToFit()
cell.textLabel!.textColor = UIColor.red
cell.textLabel!.text = self.names[uuid]
cell.textLabel!.font = UIFont.systemFont(ofSize: 20)
cell.detailTextLabel!.text = uuid.description
cell.detailTextLabel!.font = UIFont.systemFont(ofSize: 12)
return cell
}
}
extension ViewController: CBCentralManagerDelegate{
func centralManagerDidUpdateState(_ central: CBCentralManager) {
print("state \(central.state)")
switch central.state {
case .poweredOff:
print("Bluetoothの電源がOff")
case .poweredOn:
print("Bluetoothの電源はOn")
centralManager.scanForPeripherals(withServices: nil)
case .resetting:
print("レスティング状態")
case .unauthorized:
print("非認証状態")
case .unknown:
print("不明")
case .unsupported:
print("非対応")
}
}
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral,
advertisementData: [String: Any], rssi RSSI: NSNumber) {
print("pheripheral.name: \(String(describing: peripheral.name))")
print("advertisementData:\(advertisementData)")
print("RSSI: \(RSSI)")
print("peripheral.identifier.uuidString: \(peripheral.identifier.uuidString)")
let uuid = UUID(uuid: peripheral.identifier.uuid)
self.uuids.append(uuid)
let kCBAdvDataLocalName = advertisementData["kCBAdvDataLocalName"] as? String
if let name = kCBAdvDataLocalName {
self.names[uuid] = name.description
} else {
self.names[uuid] = "no name"
}
self.peripherals[uuid] = peripheral
tableView.reloadData()
}
}
Reference
- CoreBluetooth
- UITableViewDataSource